Hi coders, I will share how to create a simple e-commerce mobile app using the Flutter framework and GetX state management library with Model-View-ViewModel (MVVM) architectural patterns in this article. By the end of this article, you’ll not only have a functional e-commerce app but also a deeper understanding of how to structure and manage your Flutter projects for scalability and maintainability.
What is Flutter/GetX Flutter Library/Model-View-ViewModel (MVVM) pattern?
Flutter is an open-source framework developed by Google for building natively compiled mobile, web, and desktop applications from a single codebase. It is primarily used for creating user interfaces (UIs) and is particularly popular for mobile app development.
GetX is a popular state management and navigation library for Flutter. GetX is designed to simplify and streamline the development process by offering a set of tools and utilities that make it easier to manage application state, handle navigation, and perform other common tasks in Flutter app development.
Model-View-ViewModel (MVVM) is a software architectural pattern used to develop user interfaces, particularly in the context of software applications. MVVM is often associated with frameworks and technologies for building graphical user interfaces (GUIs) such as WPF (Windows Presentation Foundation), :, and others. It aims to separate the concerns of an application’s user interface into three distinct components which are Model, View, and ViewModel.
App Features
- Display list products.
- Display list products by category.
- Display product detail.
- Add product to Cart by quantity.
- Remove product from Cart.
The Goals
- Implementing GetX state management and GetX route in the app.
- Implementing MVVM pattern by data model (model), screen (view), and controller & binding (view-model).
- Separate screen widgets into reusable components.
- Implement the app features.
The Good Things
- Flutter Widgets system gives more flexibility, able to combine multiple widgets.
- GetX is simple, and its state & navigation management is easy to use. They have multiple ways to render the data into the view.
- A lot of Flutter packages are available and have good documentation. Useful website for Flutter package detailing ➝ https://fluttergems.dev/.
- With GetX, we can initialise multiple controllers. With this feature, we can define a global controller and screen controller and then use them for our purpose.
The Challenges
- When dealing with API requests, it's essential to establish the model according to the API response. Initially, I manually crafted the model, but later discovered helpful tools like https://javiercbk.github.io/json_to_dart/ to convert JSON responses into models, saving me valuable time.
- Occasionally, when relocating widgets, I find myself copying and pasting code, leading to errors when I forget to include the closing bracket.
App Demo:
Steps
Let's start by outlining the step-by-step process of building a basic e-commerce Mobile App using Flutter & GetX with the MVVM Pattern.
Create Flutter Project
Run the flutter create command: flutter create simple_ecommerce
If you encounter a "command not found" error, please consult this solution for resolution: https://stackoverflow.com/a/50653621.
Alternatively, you can create a Flutter project from Visual Studio Code by following this guide: https://docs.flutter.dev/get-started/test-drive?tab=vscode.
Upon successful creation, the project structure will resemble the following:
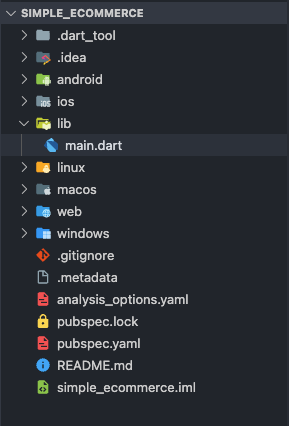
Install GetX Flutter Package
flutter pub add get
Update Project Structure
Here is project structure that we will use in this app:


Create Home Screen
There are 4 components used in the screen:
- App Bar
- Carousel
- Category Card
- Product Card
- Paginator
Let’s start creating those components:
App Bar Component
- Install “flutter svg” package https://pub.dev/packages/flutter_svg/install
flutter pub add flutter_svg
- Download cart & arrow back icon https://boxicons.com/ , then copy and paste it into ‘assets/images’. Finally, add these lines to your ‘pubspec.yaml’
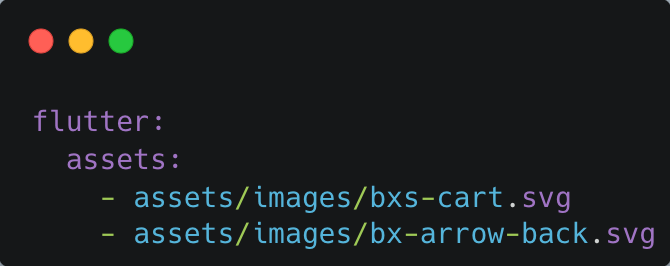
- Create a file on “lib/config/spacing.dart” and copy the code below

- Create a file on “lib/app/widgets/custom_app_bar.dart” and copy the code below
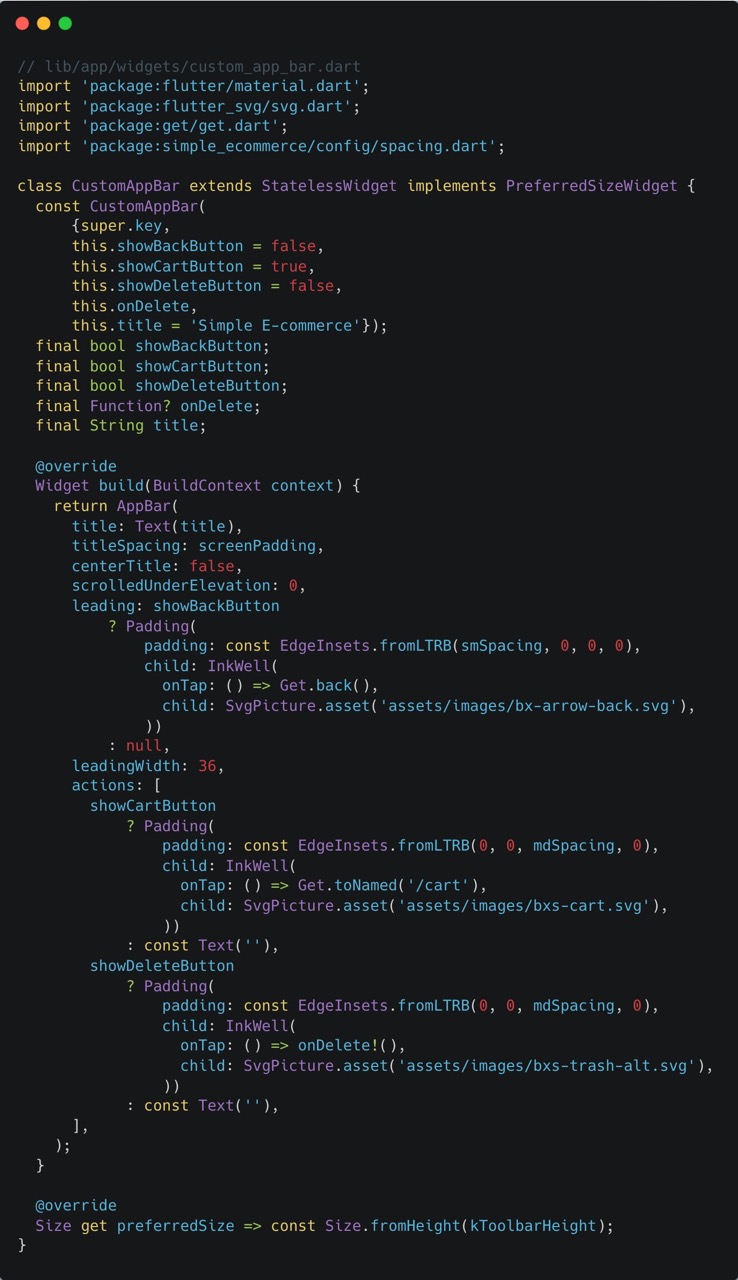
Carousel Component
- Install “carousel slider” package https://pub.dev/packages/carousel_slider
flutter pub add carousel_slider
- Install “smooth indicator” package https://pub.dev/packages/smooth_page_indicator
flutter pub add smooth_page_indicator
- Install “cached network image” package https://pub.dev/packages/cached_network_image/example
flutter pub add cached_network_image
- Create a file on “lib/app/widgets/carousel.dart” and copy the code below
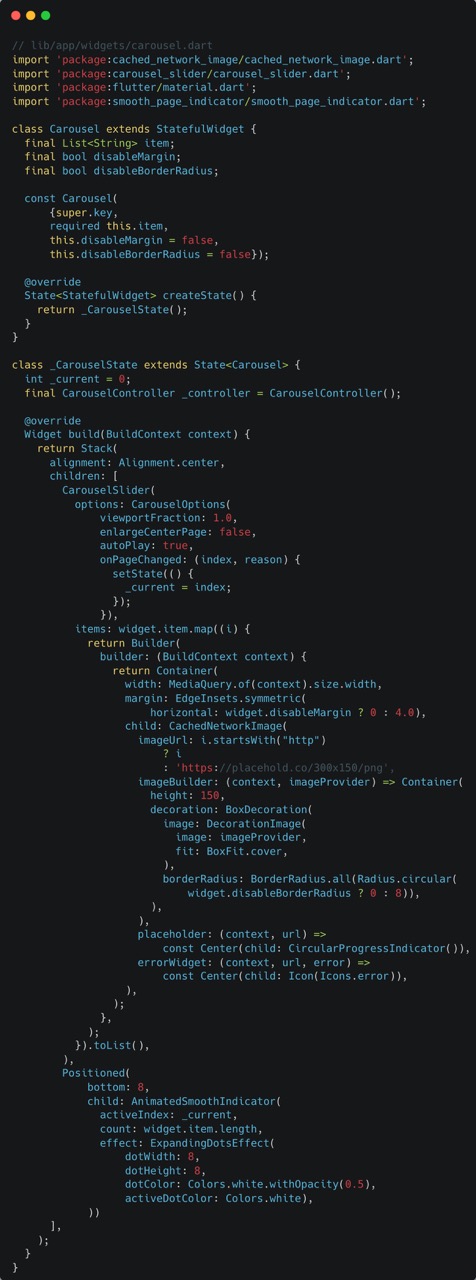
Category Card Component
Create a file on “lib/app/widgets/category_card.dart” and copy the code below

Product Card Component
Create a file on “lib/app/widgets/product_card.dart” and copy the code below

Paginator Component
Install “pagination flutter” package https://pub.dev/packages/pagination_flutter
flutter pub add pagination_flutter
Create a file on “lib/app/widgets/paginator.dart” and copy the code below:
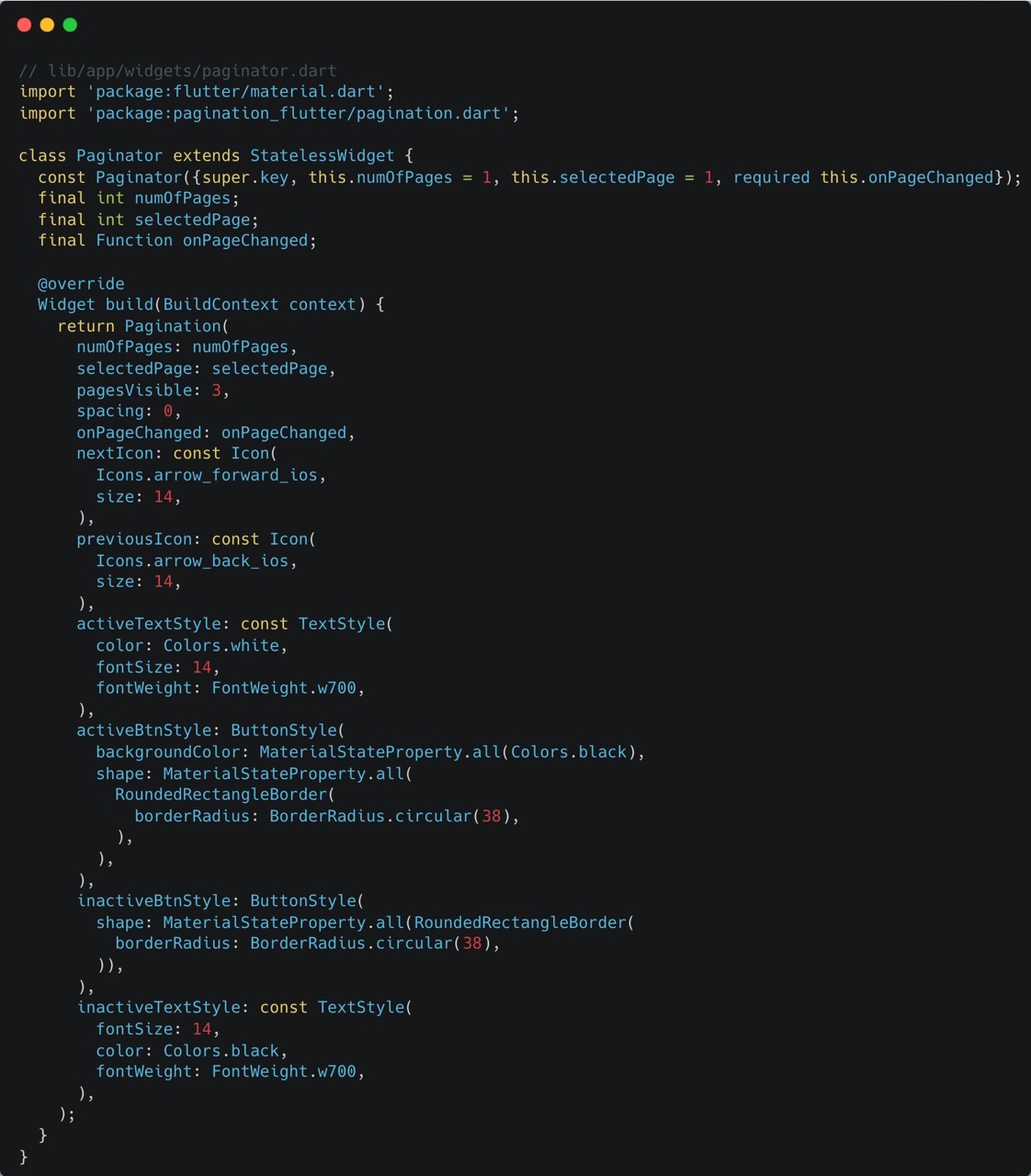
We've completed developing the Home Screen components. Now, let's delve into data modelling, API requests, and integrate everything into a single screen.
Create Model for Product
For this project, we will use fake store API to fetch the data https://fakeapi.platzi.com/. First, we need to create model for the product
Create a file on “lib/app/models/product_model.dart” and copy the code below
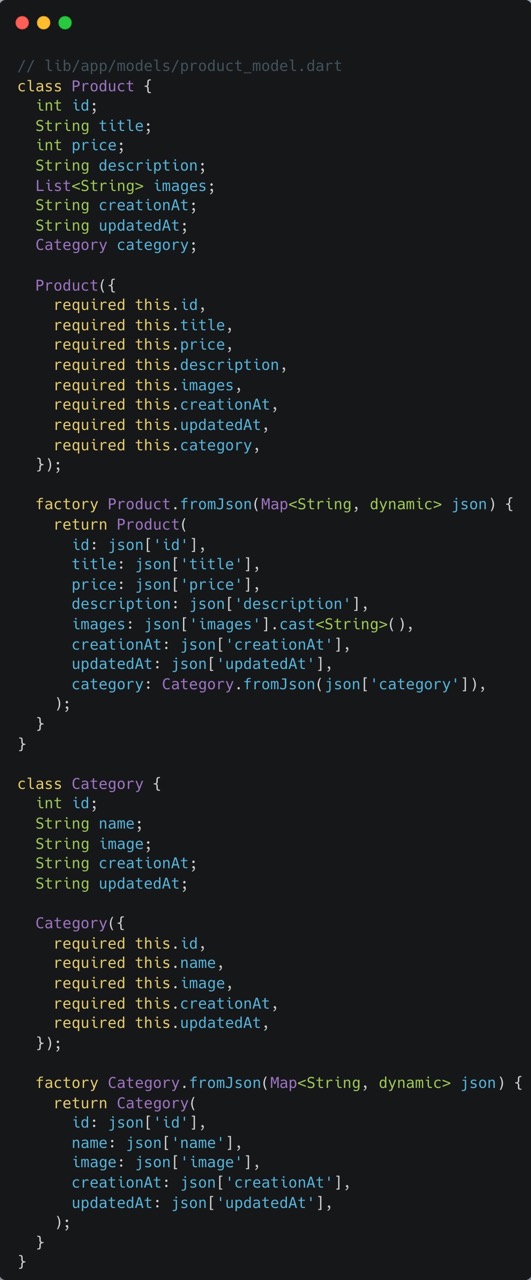
Create General Config File
Create a file on “lib/config/general.dart” and copy the code below:

Create Product Repository (API Services)
Create a file on “lib/app/repositories/product_repository.dart” and copy the code below
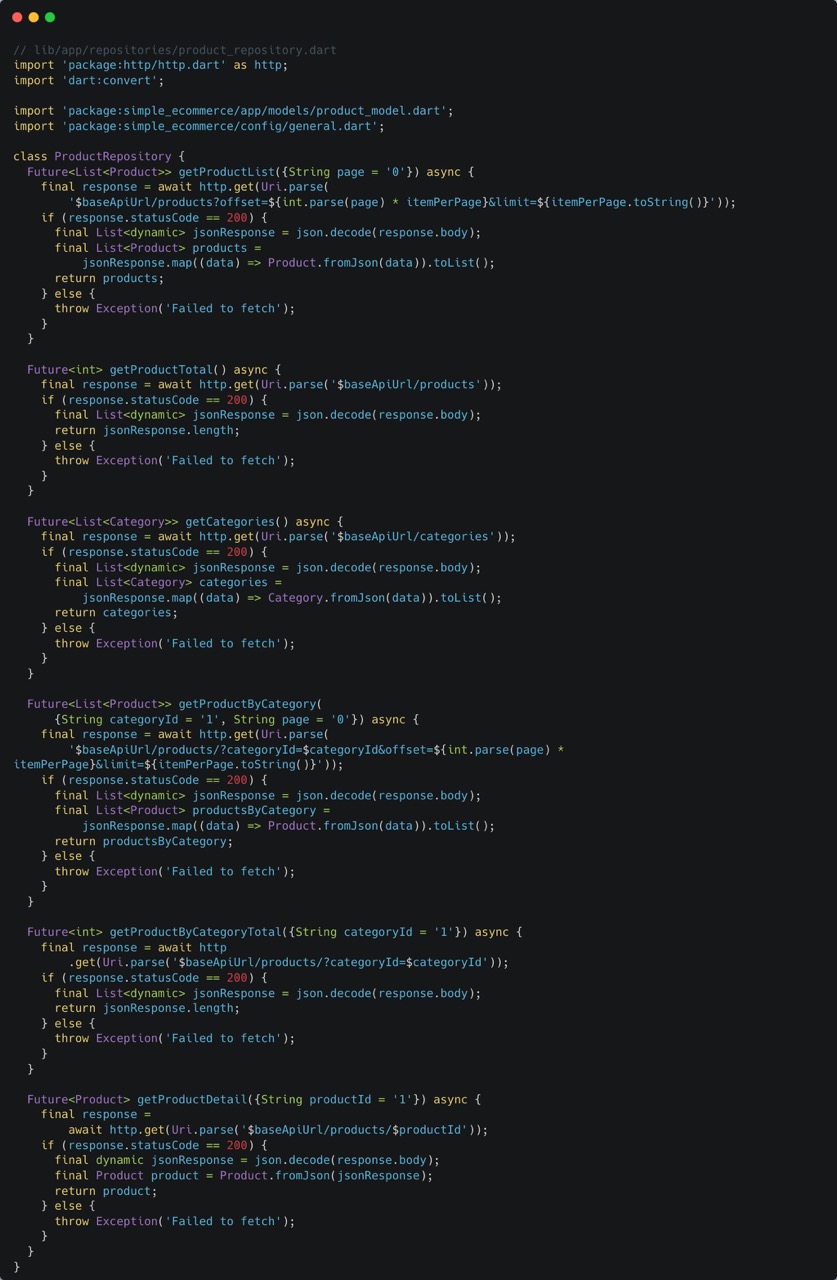
Create Home Screen Controller
Create a file on “lib/app/controllers/home_screen_controller.dart” and copy the code below:
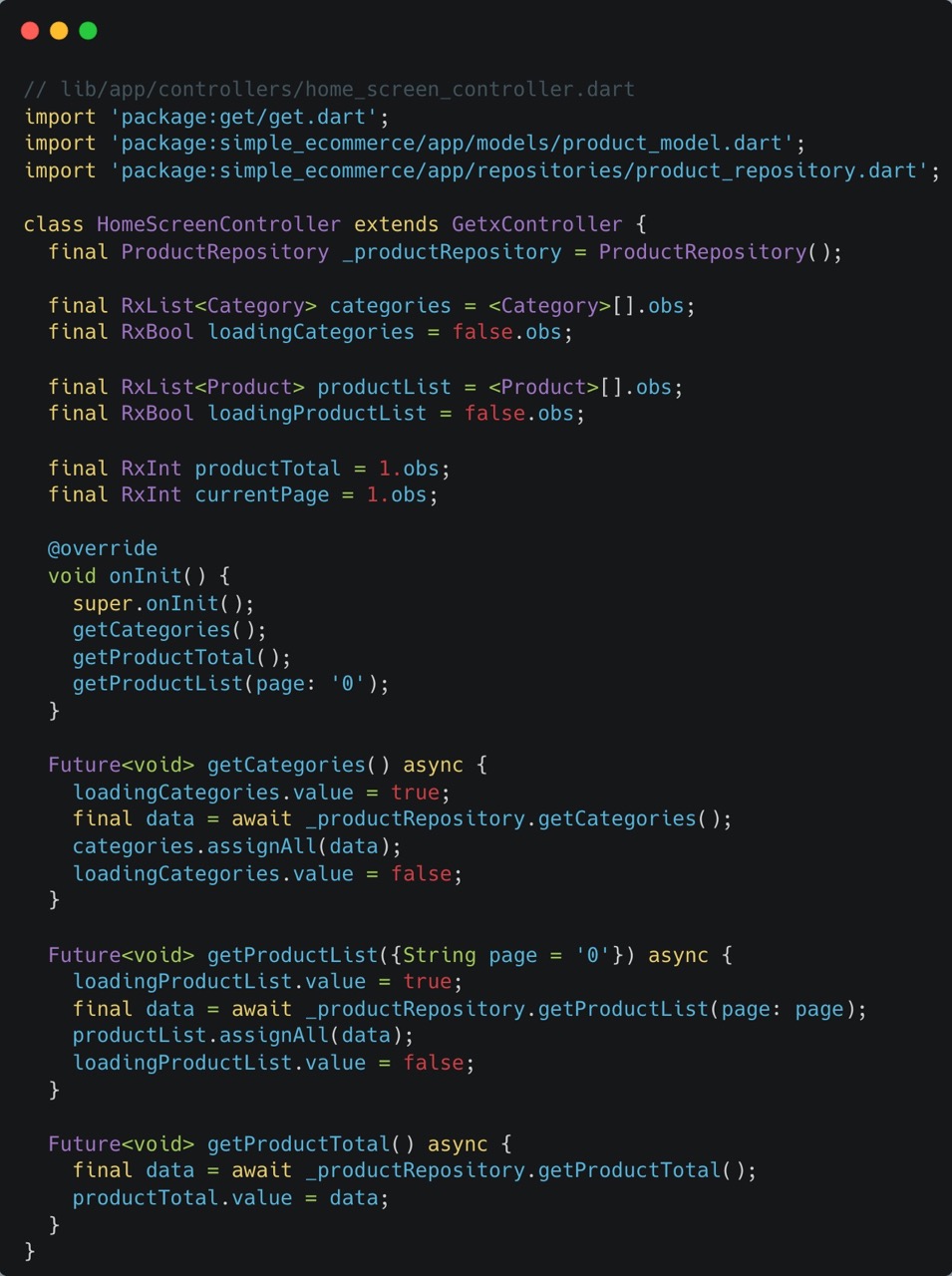
Create Home Screen Binding
Create a file on “lib/app/bindings/home_screen_binding.dart” and copy the code below

Create Home Screen View
- Install “dynamic height grid view” package https://pub.dev/packages/dynamic_height_grid_view
flutter pub add dynamic_height_grid_view
- Create a file on “lib/app/widgets/screen_padding.dart” and copy the code below

- Create a file on “lib/app/screens/home_screen.dart” and copy the code below:
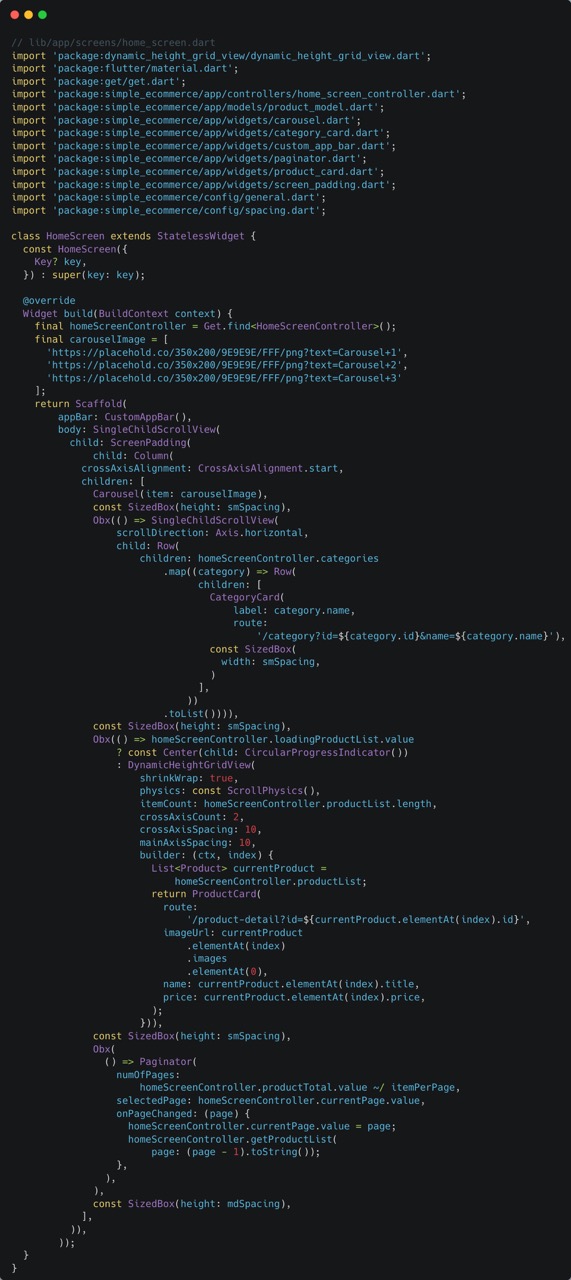
Create Category Screen
Create Category Screen Controller
Create a file on “lib/app/controllers/category_screen_controller.dart” and copy the code below:

Create Category Screen Binding
Create a file on “lib/app/bindings/category_screen_binding.dart” and copy the code below:
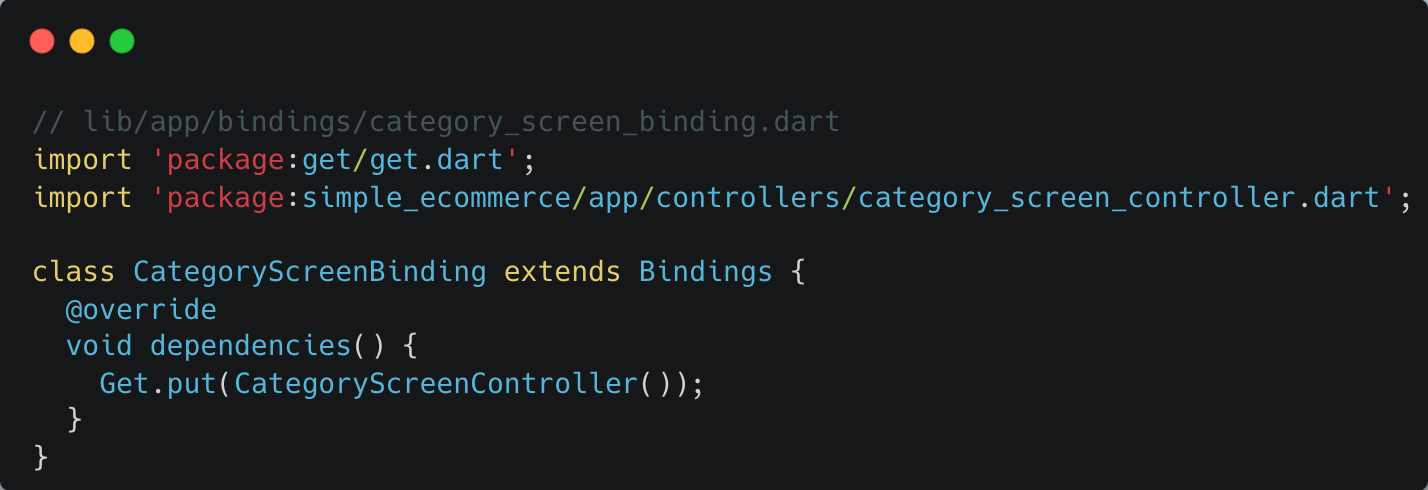
Create Category Screen View
Create a file on “lib/app/screens/category_screen.dart” and copy the code below:
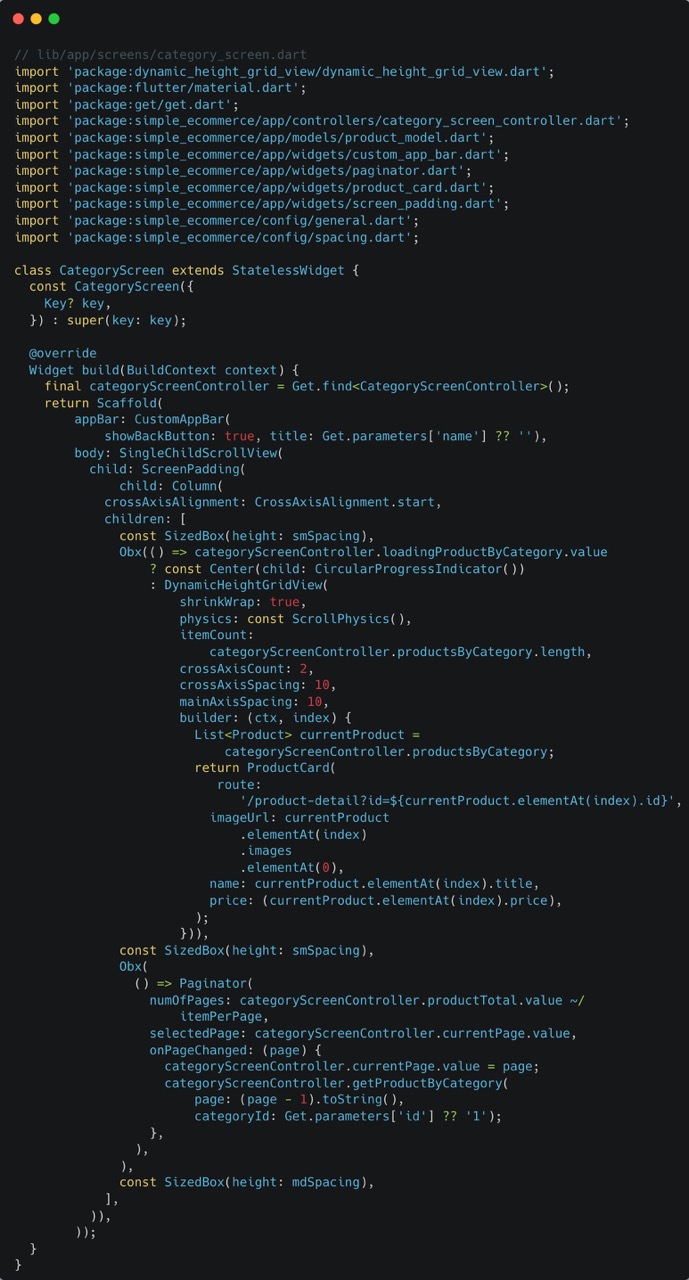
Create Product Detail Screen
Create Product Detail Screen Controller
Create a file on “lib/app/controllers/product_detail_screen_controller.dart” and copy the code below:
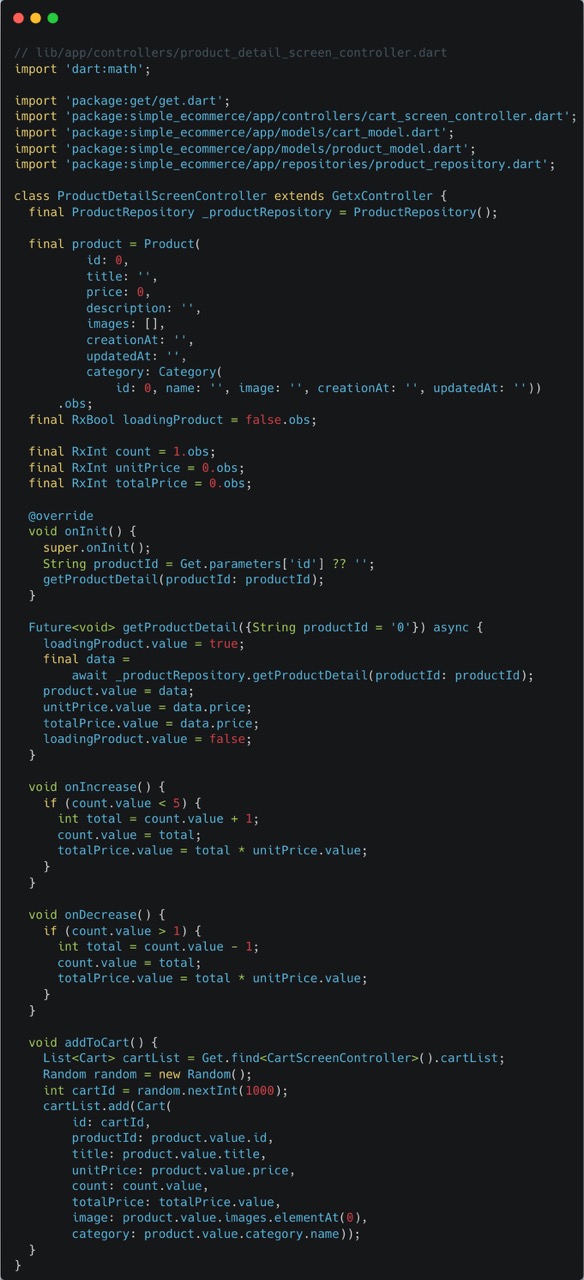
Create Product Detail Screen Binding
Create a file on “lib/app/bindings/product_detail_screen_binding.dart” and copy the code below:

Create Product Detail Screen View
Create a file on “lib/app/screens/product_detail_screen.dart” and copy the code below:
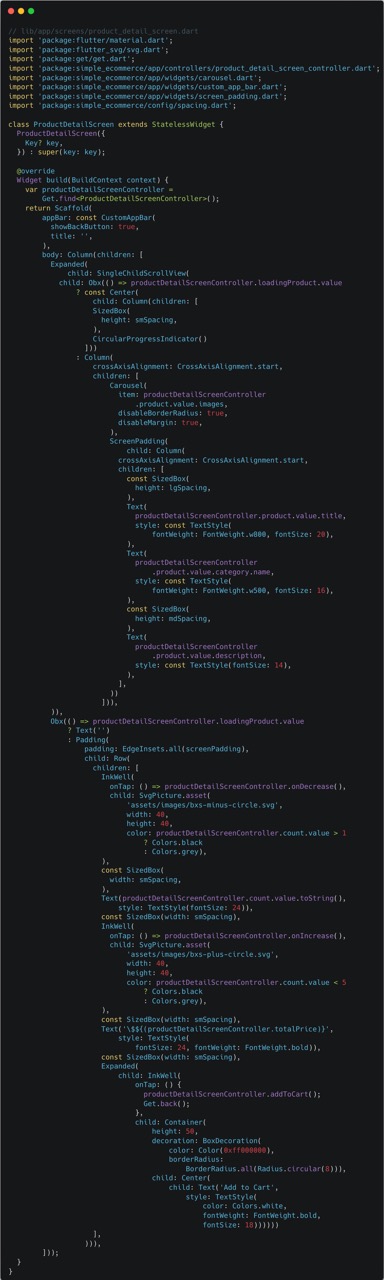
Create Cart Screen
Create Cart Model
Create a file on “lib/app/models/cart_model.dart” and copy the code below:

Create Cart Screen Controller
Create a file on “lib/app/controllers/cart_screen_controller.dart” and copy the code below:

Create Init Binding
Create a file on “lib/app/bindings/init_binding.dart” and copy the code below:

Create Cart Screen View
- Create Cart Card component on “lib/app/widgets/cart_card.dart” that will be used in Cart Screen:

Create Cart Screen view on “lib/app/screens/cart_screen.dart” and copy the code below:
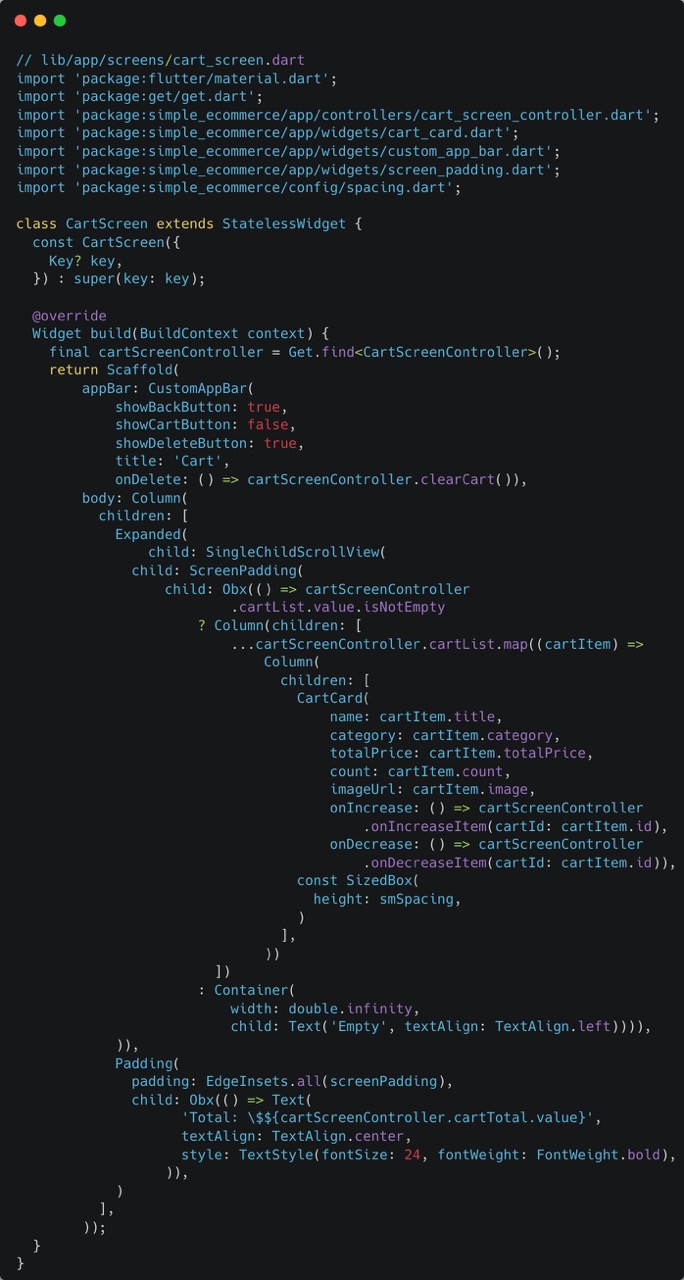
Update main.dart file
Copy the code below and replace into “lib/main.dart”:

Run the project.
Summary
This tutorial walks you through creating a straightforward e-commerce mobile app using Flutter framework and GetX state management library following the Model-View-ViewModel (MVVM) architectural pattern. Key features of the app include product display, category filtering, product detail viewing, and cart management (adding/removing products). Objectives encompass incorporating GetX state management and routing, implementing MVVM pattern, modularising screen widgets into reusable components, and realising essential app functionalities. The tutorial underscores benefits like Flutter widget flexibility, GetX simplicity, Flutter package availability, and controller setup ease. Challenges discussed entail defining models for API requests and handling widget placement errors.
Are you looking for mobile app development? We can help, let's chat.
Written by:
Widhi Mahaputra [Developer at 42 Interactive]
- As a part of 42 Interactive training and research programme -
References
https://fluttergems.dev/settings-ui/
https://github.com/jonataslaw/getx
https://fluttergems.dev/settings-ui/
https://medium.com/flutter-community/the-flutter-getx-ecosystem-state-management-881c7235511d